This is part two and final part of the blog post where we integrate firebase into react native android project and send push notification. If you have not yet created your firebase project and downloaded your google-services.json file then start by reading part one of the series. In this part, we will integrate firebase into our react native project and will send test push notification from firebase console.
Step 1: Open your react native project and install react-native-firebase by running following npm commands
npm i react-native-firebase
react-native link react-native-firebase
The first command adds the react-native-firebase package to the projects and the second command links it. Follow the next steps carefully.
Step 2: Make sure you have added google-services.json file to android->app folder as shown below. If you have not yet created google-services.json file yet read part one.
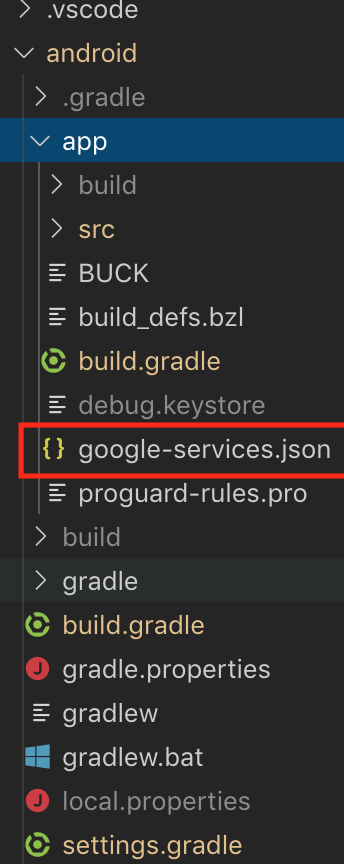
Step 3 Open your android-> build.gradle i.e project level build.gradle file. It should have dependencies looking like below
dependencies { classpath("com.android.tools.build:gradle:3.4.1") classpath 'com.google.gms:google-services:4.2.0' //<---this line should be added as shown while creating android app in firebase // NOTE: Do not place your application dependencies here; they belong // in the individual module build.gradle files }
Further below in the same build.gradle file see that allprojects looks something like this
allprojects { repositories { mavenLocal() google() . <-Make sure google() is added above jcenter() jcenter() maven { url("$rootDir/../node_modules/react-native/android") } maven { url("$rootDir/../node_modules/jsc-android/dist") } } }
Close the file we are done here. IMPORTANT we edited project level build.gradle file.
Step 4: Open app level build.gradle file from android->app->build.gradle. make sure dependencies contain the following implementation
dependencies {
implementation project(':react-native-firebase') //<-should be there already
...
implementation "com.google.android.gms:play-services-base:17.1.0"
implementation "com.google.firebase:firebase-core:17.2.0"
implementation "com.google.firebase:firebase-messaging:20.0.0"
//search for correct version number online if you encounter error
...
}
Now add the code given below at the very bottom of your build.gradle file
apply plugin: 'com.google.gms.google-services'
We are done with project-level build.gradle file. Close it.
Step 5: Now open your MainApplication.java file it is located at android->app->src->main->java->com->->MainApplication.java. In MainApplication.java file make sure imports contain following
import io.invertase.firebase.RNFirebasePackage; //<-should be there already import io.invertase.firebase.messaging.RNFirebaseMessagingPackage; import io.invertase.firebase.notifications.RNFirebaseNotificationsPackage;
Once we have imported the necessary package we need to add Firebase Messaging and notification package to package list. Make sure your get packages method adds following packages
protected List<ReactPackage> getPackages() { @SuppressWarnings("UnnecessaryLocalVariable") List<ReactPackage> packages = new PackageList(this).getPackages(); // Packages that cannot be autolinked yet can be added manually here, for example: packages.add(new RNFirebaseMessagingPackage());//added packages.add(new RNFirebaseNotificationsPackage());//added return packages; }
The two RNFirebaseMessagingPackage & RNFirebaseNotificationsPackage are not core package of react-native-firebase and hence needs to add manually. Save the file.
Step 6: Check your android-> settings.gradle file it should contain the following lines already. Else add them.
include ':react-native-firebase' project(':react-native-firebase').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-firebase/android')
Save all the edited files and run the project preferably on a device. Now you can open your firebase console and send a test notification.
Hope you guys enjoyed.