A structure that computes views on demand from an underlying collection of identified data.
Apple documents
Many a time we need to create views based on data collections. In SwiftUI we can create views in a loop using ForEach. ForEach is a view structure i.e you can use it directly in the body. Example code is given below
/ // ContentView.swift // ForEachSwiftUI // // Created by amarendra on 18/02/22. // import SwiftUI struct Student { let id = UUID() let rollnumber: Int let name: String } struct ContentView: View { let sectionA = [ Student(rollnumber: 1, name: "Aron"), Student(rollnumber: 2, name: "Betty"), Student(rollnumber: 3, name: "Clara") ] var body: some View { VStack { // id: takes a unique identifier property of element of collection to keep track of updation or deletion ForEach(sectionA, id: \.id) { student in Text("Name: \(student.name) Roll:\(student.rollnumber)") } } } } struct ContentView_Previews: PreviewProvider { static var previews: some View { ContentView() } }
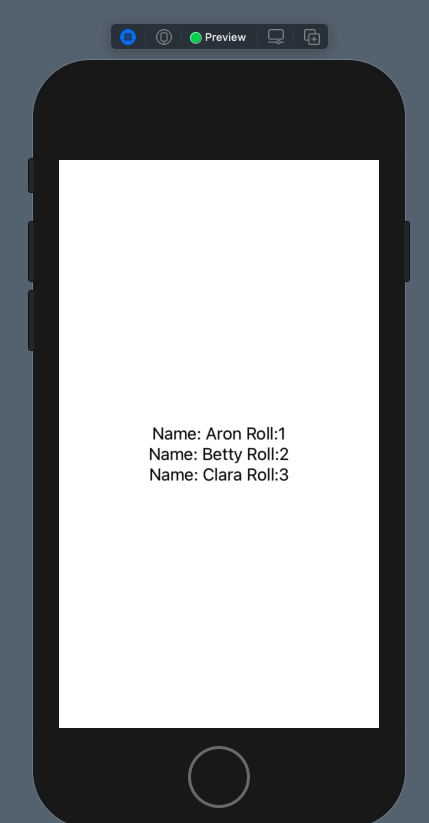
Alternatively, we can make our Student struct conform to identifiable
and then we need not explicitly pass id:
Example code is given below
// // ContentView.swift // ForEachSwiftUI // // Created by amarendra on 18/02/22. // import SwiftUI // make struct conform to Identifiable struct Student:Identifiable { let id = UUID() let rollnumber: Int let name: String } struct ContentView: View { let sectionA = [ Student(rollnumber: 1, name: "Aron"), Student(rollnumber: 2, name: "Betty"), Student(rollnumber: 3, name: "Clara") ] var body: some View { VStack { // no need to pas id:\.id ForEach(sectionA) { student in Text("Name: \(student.name) Roll:\(student.rollnumber)") } } } } struct ContentView_Previews: PreviewProvider { static var previews: some View { ContentView() } }
Hope it helps!!!