I have created a basic Ludo game with flutter which runs on both iOS and Android without any UI glitches. The base is free to use and is released under MIT license. Source code is here but read along to understand what it does.
Capabilities and Limitations
- Tokens can come out of Home Area only when dice has 6 ✔
- True Dice ✔
- Cut/Token Kill logic implemented which might not be perfect ✔
- User can tap on a token to make it move. Only valid moves are made ✔
- Safe area “Star” implemented. ✔
- Safe area coloured path near-final destination implemented. ✔
- Tokens follow the only eligible path ✔
- Animated reversal of cut/killed tokens ✔
- Works on both iOS and Android ✔
- User turn logic not implemented yet ✖
- No server-side implementation ✖
- Complex cut logics missing ✖
This is just a basic implementation which will make you realise how easy it is to make a Ludo app for both iOS and Android using flutter. Let me explain the basic game logic in details
Board Logic
Ludo board is a very simple board. It is a 15×15 (minimum usable cells) board in which only fixed cells are used for example if we talk about Green tokens the can be at only fixed number of cells which are addressable as
//GREEN home positions Token(TokenType.green, Position(2, 2), TokenState.initial, 0), Token(TokenType.green, Position(2, 3), TokenState.initial, 1), Token(TokenType.green, Position(3, 2), TokenState.initial, 2), Token(TokenType.green, Position(3, 3), TokenState.initial, 3), //All possible cells to which green can move to static const greenPath = [[6,1],[6,2],[6,3],[6,4],[6,5], [5,6],[4,6],[3,6],[2,6],[1,6],[0,6],[0,7] ,[0,8],[1,8],[2,8],[3,8],[4,8],[5,8], [6,9],[6,10],[6,11],[6,12],[6,13],[6,14], [7,14],[8,14],[8,13],[8,12],[8,11],[8,10],[8,9], [9,8],[10,8],[11,8],[12,8],[13,8],[14,8],[14,7],[14,6], [13,6],[12,6],[11,6],[10,6],[9,6], [8,5],[8,4],[8,3],[8,2],[8,1],[8,0], [7,0],[7,1],[7,2],[7,3],[7,4],[7,5],[7,6]];
There is no way a green token can end up in any other cell in a valid move. Its home position is fixed and its path is fixed. Similarly for RED, YELLOW, BLUE.
VALID MOVE LOGIC
- A move is valid if it ends within its path array
- The token is not at the final destination
- If the token is at home then it can only accept 6 as a valid move.
CUT/Kill logic
These rules might not be acceptable as is feel free to change them
- Any token which ends up in a preoccupied cell cuts/kills any different colour token already present.
- Two tokens if on the same cell create mutual safety
- There is an issue here, what happens if two tokens create safety then one other colour token arrives on top of the two tokens and in later moves one of the two same coloured Stokes has to move?
- Star cells are safety zone
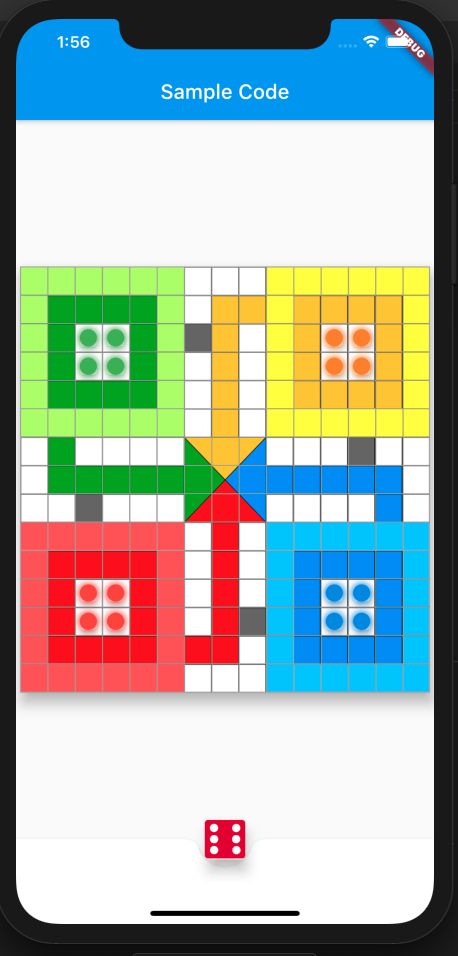
Game Play
As mentioned above, turn logic has not been implemented, neither is any ai present but you can use this app as a starting point. In order to play the game, you need to first run it using a simulator or device.
- Tap on dice at the bottom
- Tap on the token
- Token moves if the move is valid
- Cut will happen when applicable.
- a cut token will traverse all the way back to home occupying the first available slot.
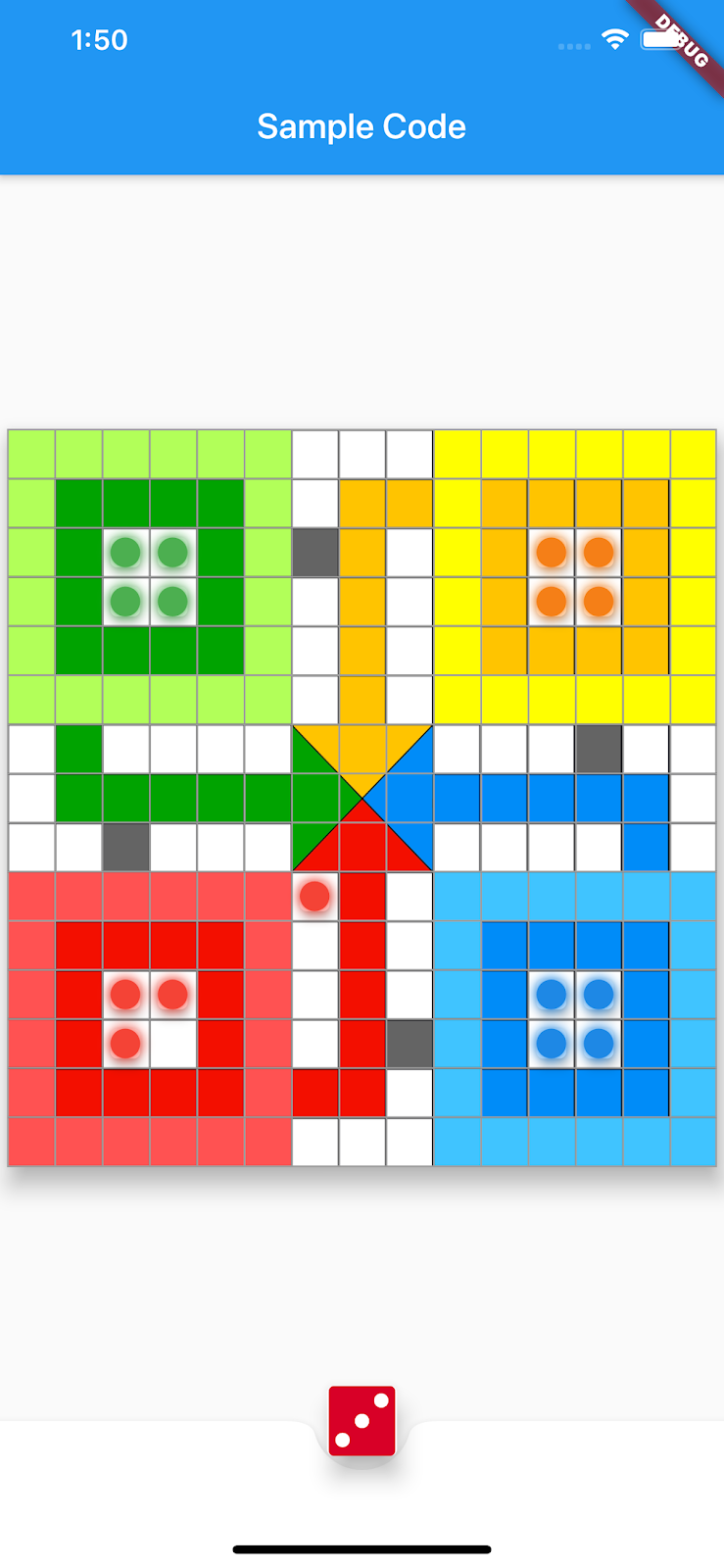
Repository : https://github.com/Amar11s/Ludo.git
Branch: prefirebase (do not use master branch)